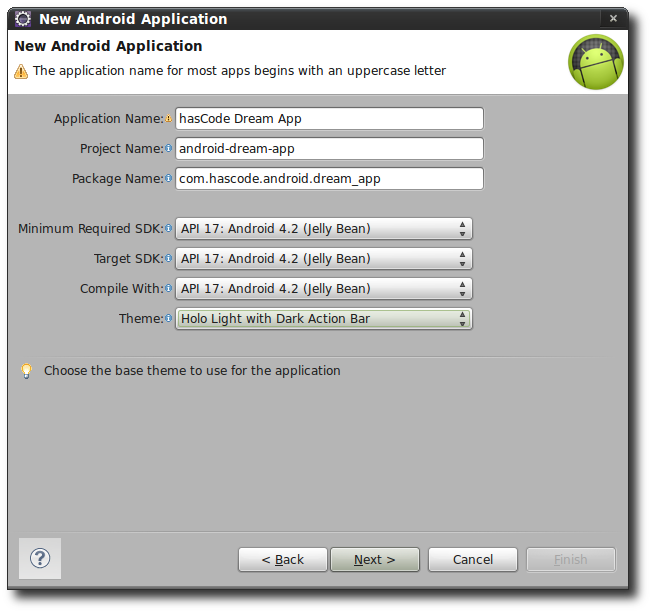
Using the Android Daydream API
Daydream is the new interactive screensaver mode that was added in the Android 4.2 / Jelly Beans release. Such “dreams” may be activated when the device is in idle mode or inserted into a dock. In the following tutorial we’re going to create a simple daydream that starts a simple animation and in addition we’ll be adding a configuration screen to alter the behaviour of this daydream. Create a new Android Application First of all we need a new android project. I am using Eclipse and the ADT plugin here but any other solution like using a specific Maven archetype and the Jayway Maven plugin or using SBT should work, too. ...