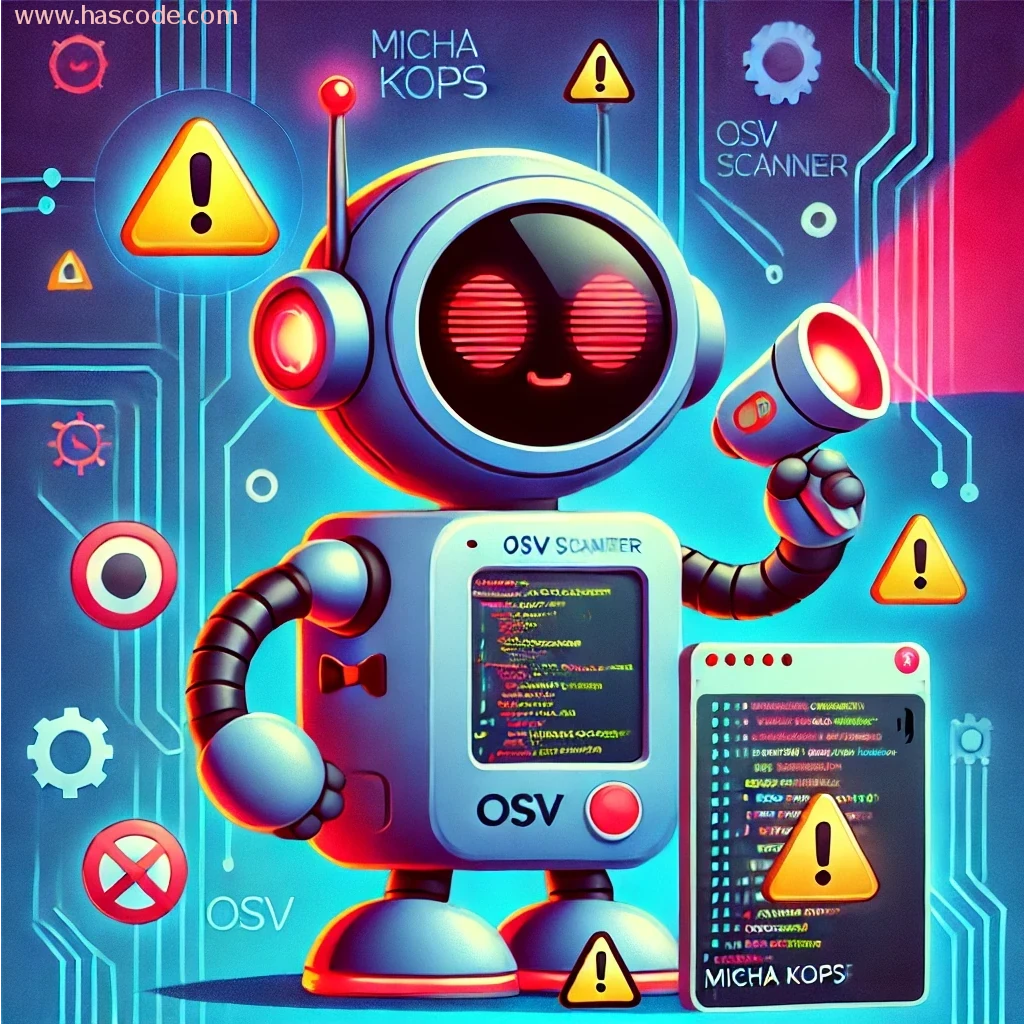
CVE Scanning and Guided Remediation with OSV Scanner
Figure 1. OSV Scanner Security is a critical aspect of software development, and staying ahead of vulnerabilities is essential for us application developers. Google’s OSV Scanner is a powerful tool that helps detect vulnerabilities in open-source dependencies. This article will guide us through setting up and using OSV Scanner to secure our projects, scan for invalid licenses, scan OCI images and finally how to fix findings via guided remediation. ...